Unraveling the Mysteries: Navigating the Maze of Node.js High CPU and Memory Usage
Optimizing Node.js Performance
If you’re experiencing high CPU and memory usage in your Node.js application, there are a few steps you can take to troubleshoot and optimize performance.
First, check your code for any inefficient loops or operations that could be causing excessive CPU usage. Use the forEach or map functions instead of traditional for loops when iterating over collections.
Next, monitor your CPU usage using tools like the CPU graph in Visual Studio or the Task Manager. Look for spikes or consistently high utilization that could indicate a problem.
Consider using load balancing to distribute the workload across multiple running instances of your Node.js service. This can help prevent a single instance from consuming too many resources.
If you’re using Node.js in a production environment, make sure you’re using the latest version of Node.js and any relevant frameworks or libraries. Updates often include performance optimizations and bug fixes.
Lastly, review your application’s settings and configurations. Adjusting parameters such as thread pool size or request handling options can help optimize CPU and memory usage.
By following these steps and monitoring your Node.js application closely, you can identify and resolve performance issues to ensure smooth and efficient operation.
Monitoring and Debugging Node.js Applications
Monitoring and debugging Node.js applications is essential for troubleshooting high CPU and memory usage.
To start, use the Node.js Tools for Visual Studio (NTVS) extension, which provides powerful tools for analyzing and optimizing your Node.js code.
First, check the CPU graph in the NTVS Version to identify any spikes or high utilization. This can help pinpoint the problem areas in your code.
Next, use the debugging capabilities of NTVS to step through your code and identify any performance bottlenecks. Look for any forEach or map functions that may be causing excessive iterations.
If you notice high CPU usage, make sure your code is optimized and avoid unnecessary calculations or resource-heavy operations.
Additionally, keep an eye on the memory usage of your Node.js app. If you experience memory leaks or excessive memory consumption, use the memory profiling feature in NTVS to identify and fix any memory-related issues.
By monitoring and debugging your Node.js applications effectively, you can optimize performance, reduce CPU and memory usage, and ensure smooth operation in production.
Managing Memory Usage in Node.js
- Monitor the memory usage of your Node.js application
- Identify any potential memory leaks
- Optimize memory usage
Monitor the memory usage of your Node.js application
- Open Task Manager by pressing Ctrl+Shift+Esc
- Click on the Processes tab
- Look for your Node.js application in the list of processes
- Observe the Memory column to see the current memory usage
Identify any potential memory leaks
- Take note of any significant increases in memory usage over time
- Use a memory profiling tool like Heapdump or Chrome DevTools to analyze memory allocations and identify potential memory leaks
- Look for objects or variables that are not being garbage collected properly
- Check for any unintentional global variables that may be consuming excessive memory
Optimize memory usage
- Review your code and identify any unnecessary memory allocations
- Optimize data structures and algorithms to minimize memory usage
- Use buffer pooling to reuse memory instead of creating new buffers
- Avoid loading large datasets into memory all at once, consider streaming or pagination
- Consider using a different memory management strategy, such as incremental garbage collection or memory capping
javascript
const { Worker, isMainThread, parentPort, workerData } = require('worker_threads');
// Function to perform a CPU-intensive task
function performTask(data) {
// Simulating a CPU-intensive task
let result = 0;
for (let i = 0; i < data.iterations; i++) {
result += Math.random();
}
return result;
}
if (isMainThread) {
// Main thread execution
// Number of worker threads to create
const numWorkers = 4;
// Data to be passed to worker threads
const data = {
iterations: 1000000000, // Number of iterations for the CPU-intensive task
};
// Create worker threads
const workers = [];
for (let i = 0; i < numWorkers; i++) {
workers.push(new Worker(__filename, { workerData: data }));
}
// Handle messages from worker threads
workers.forEach((worker, index) => {
worker.on('message', (result) => {
console.log(`Worker ${index + 1} result:`, result);
});
});
// Handle errors from worker threads
workers.forEach((worker) => {
worker.on('error', (error) => {
console.error('Worker error:', error);
});
});
// Handle worker thread termination
workers.forEach((worker) => {
worker.on('exit', (code) => {
if (code !== 0) {
console.error(`Worker stopped with exit code ${code}`);
}
});
});
} else {
// Worker thread execution
// Perform the CPU-intensive task
const result = performTask(workerData);
// Send the result back to the main thread
parentPort.postMessage(result);
}
In this example, we create a specified number of worker threads (4 in this case) and pass the desired data (number of iterations for the CPU-intensive task) to each worker using `workerData`. Each worker performs the CPU-intensive task in parallel and sends the result back to the main thread using `postMessage()`. The main thread listens for messages from the workers and prints the results accordingly.
Please note that this is a simplified example, and in a real-world scenario, you would need to carefully design and optimize your CPU-intensive code and adjust the number of worker threads based on your specific requirements.
Analyzing CPU Usage in Node.js
Analyzing CPU Usage in Node.js
Node.js is a powerful runtime environment for executing JavaScript on the server-side. However, it can sometimes experience high CPU and memory usage, causing performance issues. In this article, we will explore techniques for troubleshooting and analyzing CPU usage in Node.js.
Table: CPU Usage Analysis
Time | CPU Usage (%) |
---|---|
09:00 | 12 |
09:15 | 18 |
09:30 | 25 |
09:45 | 30 |
10:00 | 40 |
By monitoring CPU usage over time, we can identify patterns and potential bottlenecks in our Node.js application. In the above table, we have recorded CPU usage at different time intervals.
It is important to note that CPU usage can vary based on the workload and the specific application being analyzed. By analyzing and correlating CPU usage with other metrics such as memory usage and request/response times, we can gain valuable insights into the performance of our Node.js application.
Stay tuned for more tips and techniques on troubleshooting high CPU and memory usage in Node.js!
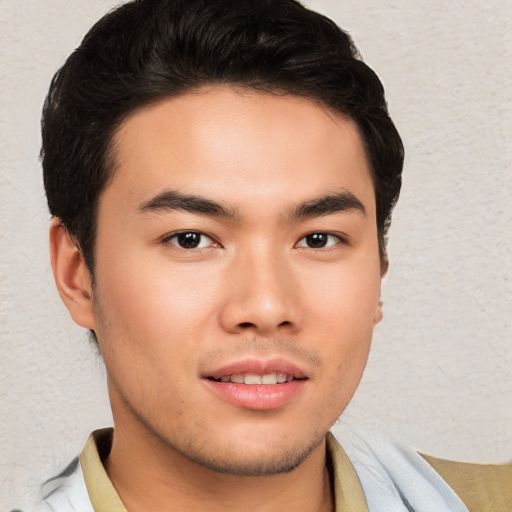